Le moyen le plus simple d'intégrer des applications à des voitures
L'API pour véhicules de Smartcar vous permet de vérifier le kilométrage, de gérer la recharge des véhicules électriques, de délivrer des clés de voiture numériques, de suivre les flottes et bien plus encore.
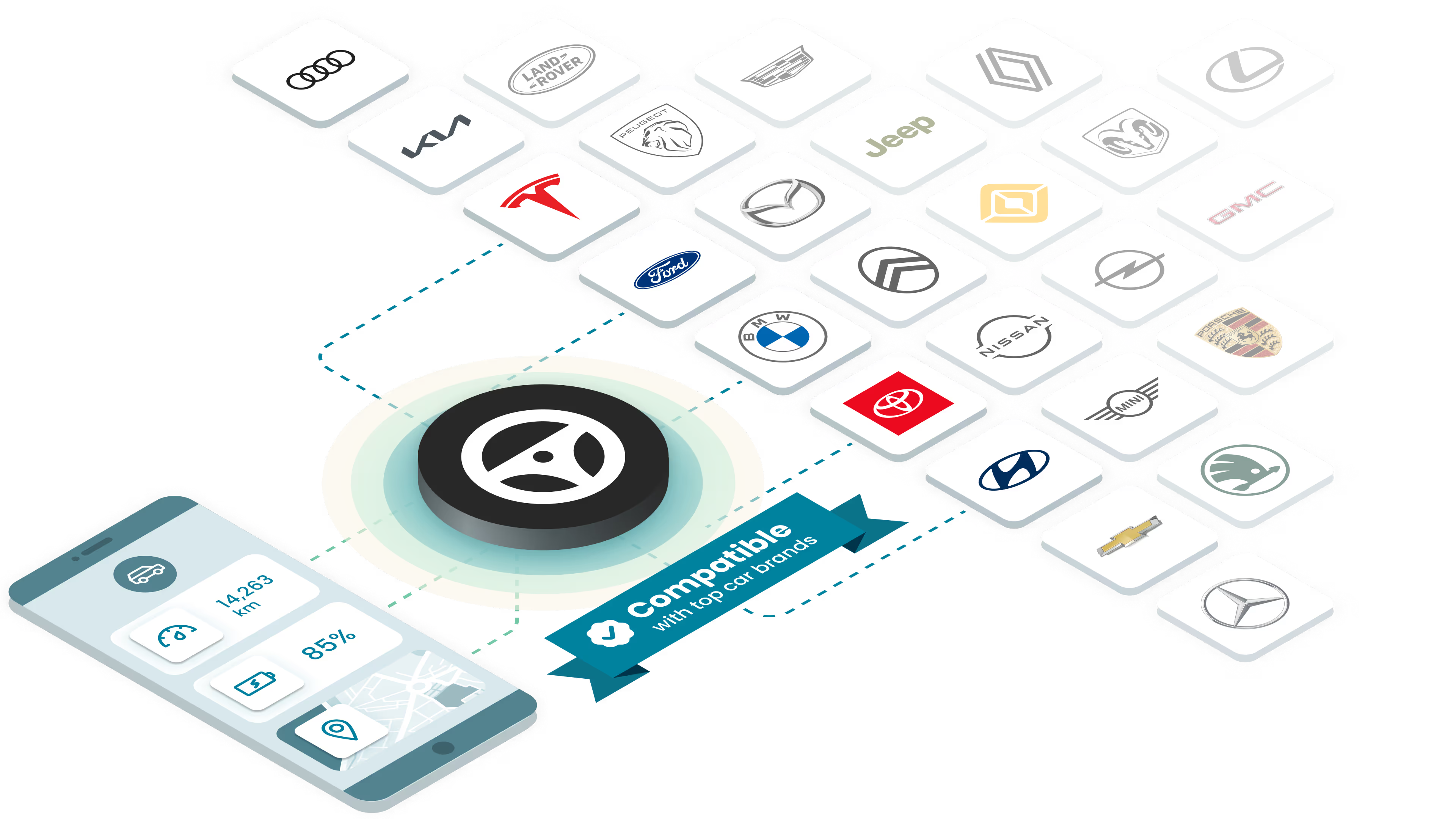
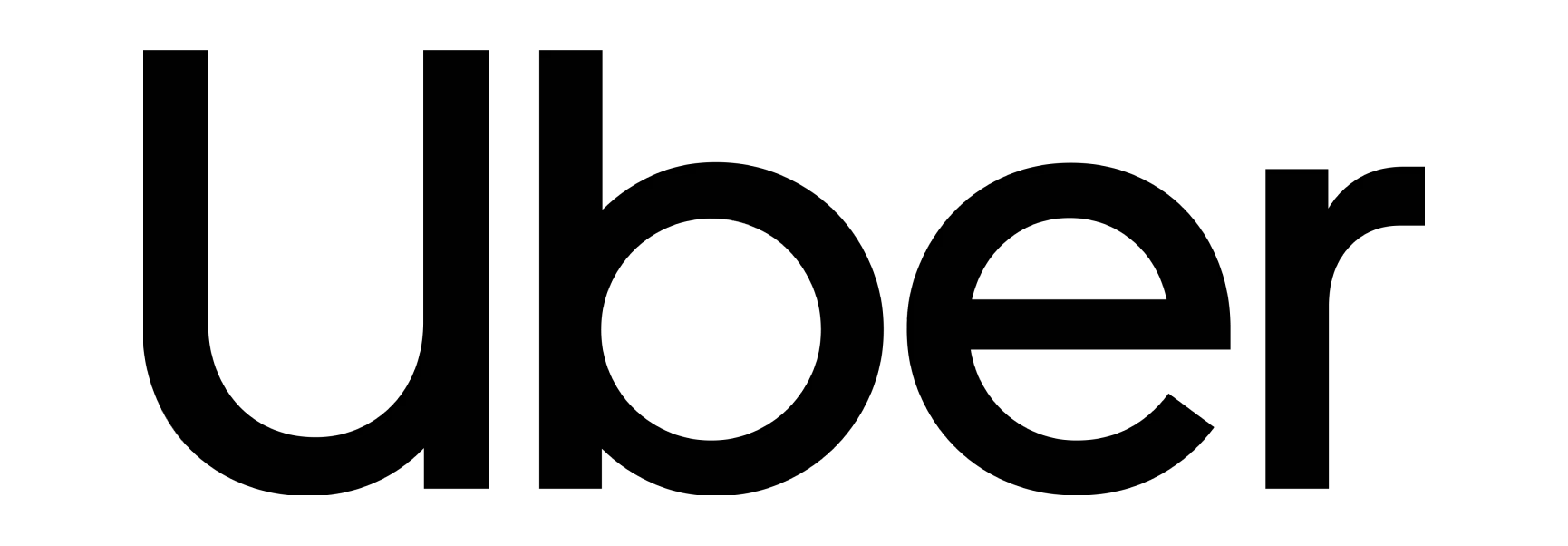
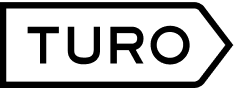

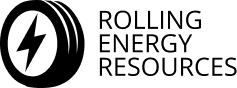

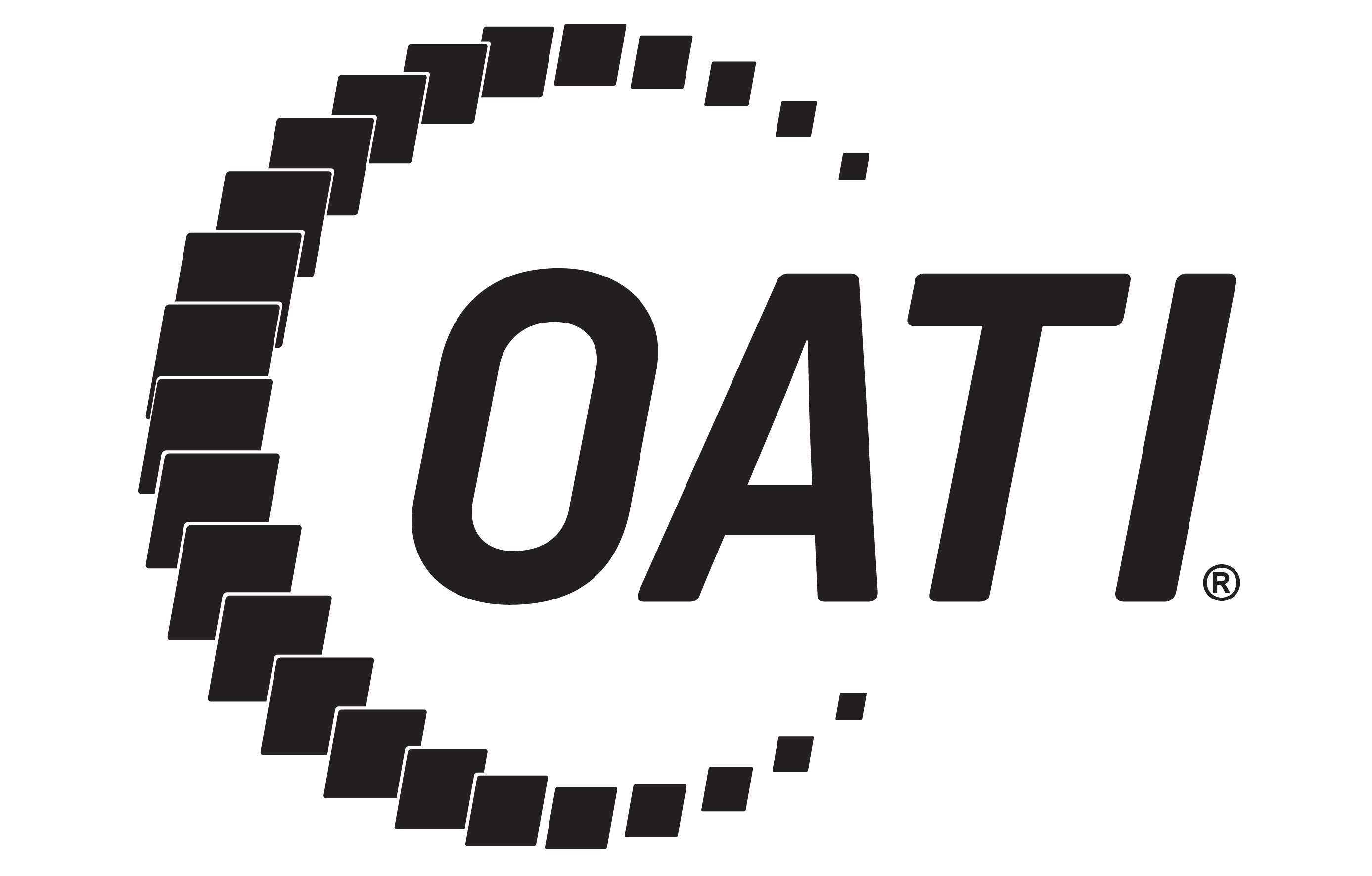

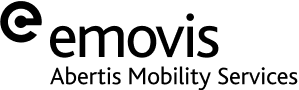

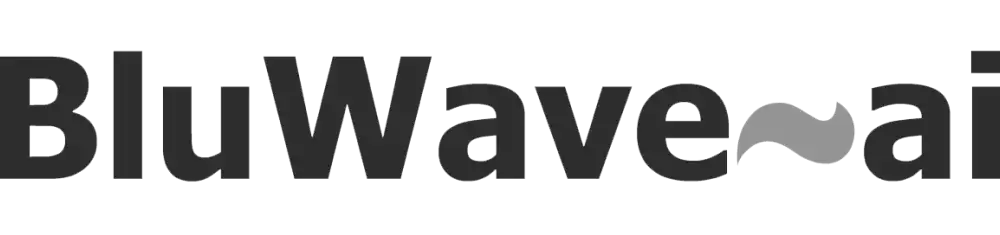
Favoriser la mobilité dans le monde
Smartcar permet aux entreprises de mobilité d'intégrer leurs applications et services aux voitures connectées. Récupérez facilement les données du véhicule et déclenchez des actions grâce à notre API automobile simple et sécurisée. Suivez la position d'un véhicule, vérifiez le kilométrage, rechargez un véhicule électrique, partagez une clé virtuelle, etc.
Intégrez les utilisateurs en quelques secondes
Grâce à notre flux Smartcar Connect, vos clients peuvent associer instantanément et sans effort leurs voitures à votre application. Il suffit de quatre clics directement depuis votre application mobile ou votre portail Web.
- Fonctionne avec votre application Web ou mobile
- Interface utilisateur simple et élégante
- Supporte l'autorisation OAuth2
- Disponible en plusieurs langues


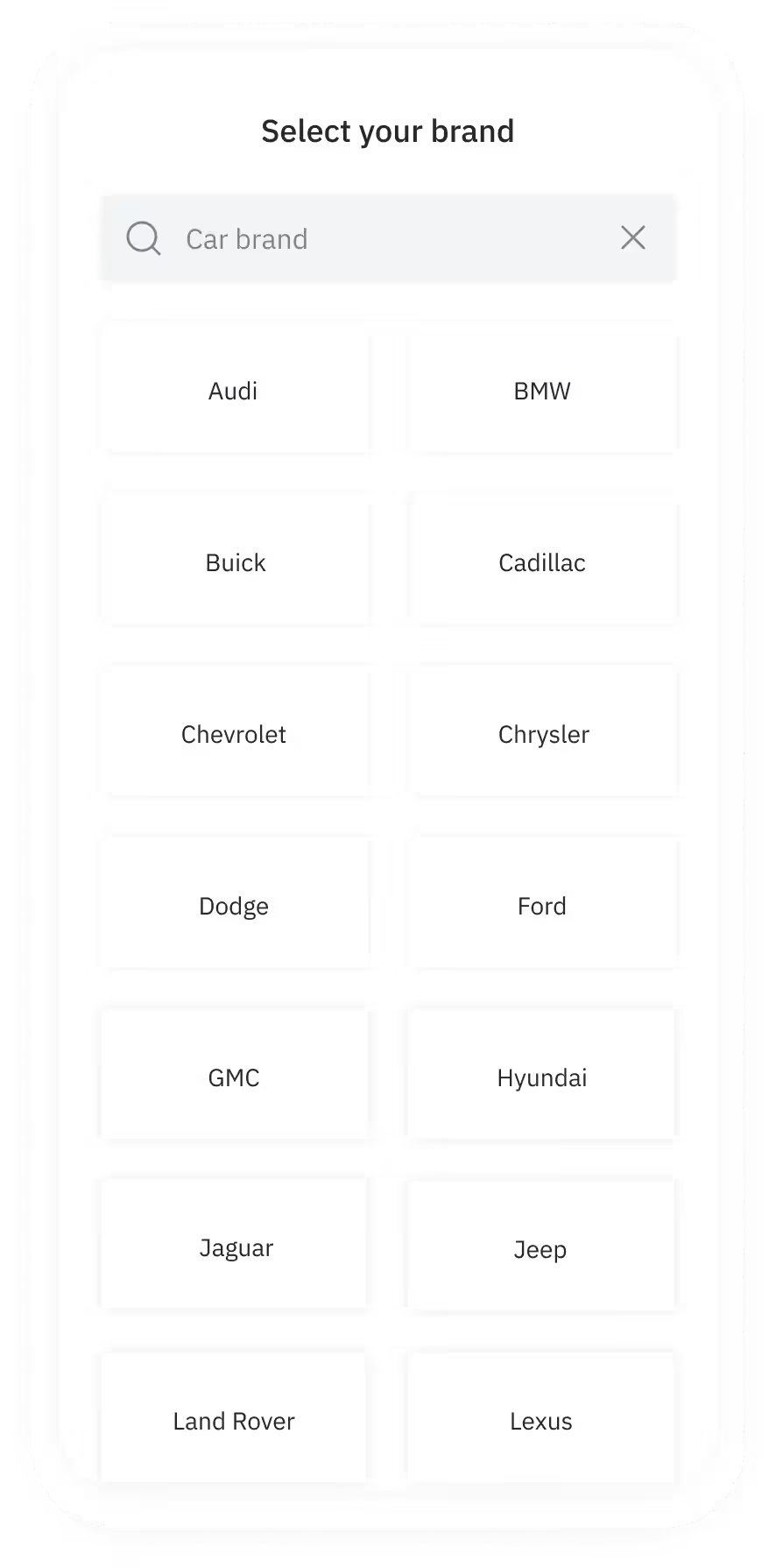



Une expérience exceptionnelle pour les développeurs
Notre documentation conviviale et nos kits de développement logiciel (SDK) vous permettent d'intégrer rapidement notre API automobile à chaque stack technologique.
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's location
const location = await vehicle.location();
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's location
location = vehicle.location()
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's location
VehicleLocation location = vehicle.location();
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's location
var fuel, resErr = vehicle.GetLocation(context.TODO());
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's location
location = vehicle.location()
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's odometer
const odometer = await vehicle.odometer();
// Example http response from Smartcar
{
"distance": 104.32
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's odometer
odometer = vehicle.odometer()
// Example http response from Smartcar
{
"distance": 104.32
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's odometer
VehicleOdometer odometer = vehicle.odometer();
// Example http response from Smartcar
{
"distance": 104.32
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's odometer
var odometer, resErr = vehicle.GetOdometer(context.TODO());
// Example http response from Smartcar
{
"distance": 104.32
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's odometer
odometer = vehicle.odometer()
// Example http response from Smartcar
{
"distance": 104.32
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Lock the vehicle
await vehicle.lock();
// Unlock the vehicle
await vehicle.unlock();
// Example http response from Smartcar
{
"status": "success"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Lock the vehicle
vehicle.lock()
# Unlock the vehicle
vehicle.unlock()
// Example http response from Smartcar
{
"status": "success"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Lock the vehicle
vehicle.lock();
// Unlock the vehicle
vehicle.unlock();
// Example http response from Smartcar
{
"status": "success"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Lock the vehicle
var lock, resErr = vehicle.Lock(context.TODO());
// Unlock the vehicle
var unlock, resErr = vehicle.Unlock(context.TODO());
// Example http response from Smartcar
{
"status": "success"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Lock the vehicle
vehicle.lock!
# Unlock the vehicle
vehicle.unlock!
// Example http response from Smartcar
{
"status": "success"
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's battery level
const battery = await vehicle.battery();
// Fetch the vehicle's battery capacity
const batteryCapacity = await vehicle.batteryCapacity();
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's battery level
battery = vehicle.battery()
# Fetch the vehicle's battery capacity
battery_capacity = vehicle.battery_capacity()
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's battery level
VehicleBattery battery = vehicle.battery();
// Fetch the vehicle's battery capacity
VehicleBatteryCapacity batteryCapacity = vehicle.batteryCapacity();
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's battery level
var battery, resErr = vehicle.GetBattery(context.TODO());
// Fetch the vehicle's battery capacity
var batteryCapacity, resErr = vehicle.GetBatteryCapacity(context.TODO());
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's battery level
battery = vehicle.battery()
# Fetch the vehicle's battery capacity
battery_capacity = vehicle.battery_capacity()
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's charging status
const charge = await vehicle.charge();
// Start the vehicle's charging session
await vehicle.startCharge();
// Set the vehicle's charge limit
await vehicle.setChargeLimit(0.8);
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's charging status
charge = vehicle.charge()
# Start the vehicle's charging session
vehicle.start_charge()
# Set the vehicle's charge limit
vehicle.set_charge_limit(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's charging status
VehicleCharge charge = vehicle.charge();
// Start the vehicle's charging session
vehicle.startCharge();
// Set the vehicle's charge limit
vehicle.setChargeLimit(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's charging status
var charge, resErr = vehicle.GetCharge(context.TODO());
// Start the vehicle's charging session
var startCharge, resErr = vehicle.StartCharge(context.TODO());
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's charging status
charge = vehicle.charge()
# Start the vehicle's charging session
vehicle.startCharge!
# Set the vehicle's charge limit
vehicle.set_charge_limit!(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's fuel tank level
const fuel = await vehicle.fuel();
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's fuel tank level
fuel = vehicle.fuel()
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's fuel tank level
VehicleFuel fuel = vehicle.fuel();
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's fuel tank level
var fuel, resErr = vehicle.GetFuel(context.TODO());
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's fuel tank level
fuel = vehicle.fuel()
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's tire pressure
const tirePressure = await vehicle.tirePressure();
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's tire pressure
tire_pressure = vehicle.tirePressure()
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's attributes
VehicleTirePressure tirePressure = vehicle.tirePressure();
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"}
);
// Fetch the vehicle's tire pressure
var tirePressure, resErr = vehicle.GetTirePressure(context.TODO());
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's tire pressure
tire_pressure = vehicle.tire_pressure()
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's engine oil life
const oil = await vehicle.oil();
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's engine oil life
oil = vehicle.oil()
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's engine oil life
VehicleEngineOil oil = vehicle.oil();
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's engine oil life
var oil, resErr = vehicle.GetOil(context.TODO());
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's engine oil life
engine_oil = vehicle.engine_oil()
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's attributes
const vehicleAttributes = await vehicle.attributes();
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's attributes
vehicle_attributes = vehicle.attributes()
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's attributes
VehicleAttributes vehicleAttributes = vehicle.attributes();
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's attributes
var info, resErr = vehicle.GetInfo(context.TODO());
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's attributes
vehicle_attributes = vehicle.attributes()
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's VIN
const vin = await vehicle.vin();
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's VIN
vin = vehicle.vin()
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's VIN
VehicleVin vin = vehicle.vin();
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's VIN
var VIN, resErr = vehicle.GetVIN(context.TODO());
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's VIN
vin = vehicle.vin()
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
XX
XX
XX
XX
Bien plus qu'une simple API de données automobiles
Grâce à notre plateforme de développement unifiée, la création et la mise à l'échelle de services de mobilité sont un jeu d'enfant.
Une API de voiture pour XX marques
Notre API pour voiture est compatible avec XX fait, permettant à votre application ou à votre service de se connecter à plus de XXM véhicules avec une seule intégration.
Donnez le contrôle à vos clients
Smartcar Connect permet à vos clients de relier leurs voitures à votre application Web ou mobile en quelques clics. Connect est le moyen le plus rapide et le plus transparent de recueillir le consentement des utilisateurs.
Sans matériel
Nos API communiquent directement avec le modem cellulaire intégré à la plupart des véhicules, sans avoir besoin de matériel de rechange tel que des dongles OBD-II.
Fiable et sécurisé
Smartcar est conforme au RGPD, à la norme ISO 27001, à la norme ISO 27701 et à la norme SOC 2 Type 2. Notre API pour voiture est cryptée avec le protocole SSL/TLS 1.2 de niveau bancaire et conforme au protocole d'autorisation OAuth2.
Ce que disent nos clients
“Smartcar is making it easier than ever for us to build new and innovative mobility experiences with connected vehicles.”
“When looking for an electric vehicle API provider, Smartcar was the only option to fit the bill. Instead of calling out to tons of different vehicle APIs, we only need to call out to one.”
“Not only does the Smartcar platform provide amazing technology, but their team also helped us integrate in just a few weeks, which enabled us to scale incredibly fast!”
“Smartcar has enabled us to level up our EV Managed Charging platform for electric utilities by filling an important technology gap — connecting to the vehicle.”
“Thanks to Smartcar's integration, our smart energy management app, ALICE, can seamlessly support over 60 EV models.”
“We chose Smartcar’s vehicle APIs to augment our EV Everywhere product capabilities, advancing our mission of promoting sustainable energy solutions for the energy and transportation sectors.”